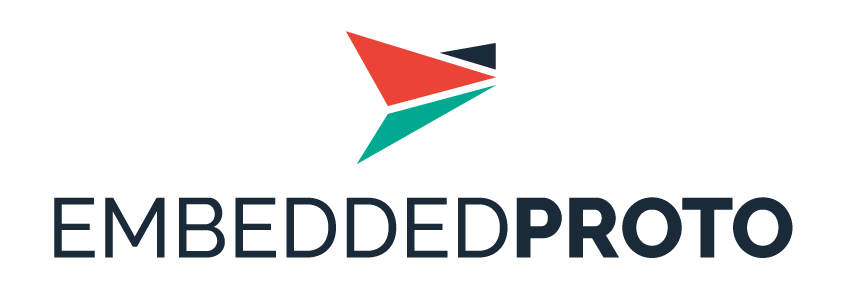 |
EmbeddedProto
2.0.0
EmbeddedProto is a C++ Protocol Buffer implementation specifically suitable for microcontrollers.
|
Go to the documentation of this file.
31 #ifndef _REPEATED_FIELD_H_
32 #define _REPEATED_FIELD_H_
42 #include <type_traits>
49 template<
class DATA_TYPE>
52 static_assert(std::is_base_of<::EmbeddedProto::Field, DATA_TYPE>::value,
"A Field can only be used as template paramter.");
55 static constexpr
bool REPEATED_FIELD_IS_PACKED =
56 !(std::is_base_of<MessageInterface, DATA_TYPE>::value
57 || std::is_base_of<internal::BaseStringBytes, DATA_TYPE>::value);
71 virtual uint32_t
get_size()
const = 0;
81 virtual DATA_TYPE&
get(uint32_t index) = 0;
88 virtual const DATA_TYPE&
get_const(uint32_t index)
const = 0;
109 virtual void set(uint32_t index,
const DATA_TYPE& value) = 0;
117 virtual Error set_data(
const DATA_TYPE* data,
const uint32_t length) = 0;
124 virtual Error add(
const DATA_TYPE& value) = 0;
127 virtual void clear() = 0;
140 if(REPEATED_FIELD_IS_PACKED)
145 if(size_x <= buffer.get_available_size())
157 return_value = serialize_packed(buffer);
170 if(size_x <= buffer.get_available_size())
172 return_value = serialize_unpacked(field_number, buffer);
192 if(REPEATED_FIELD_IS_PACKED)
194 return_value = deserialize_packed(buffer);
198 return_value = deserialize_unpacked(buffer);
211 serialize_packed(calcBuffer);
222 serialize_unpacked(field_number, calcBuffer);
233 return_value = this->
get_const(i).serialize(buffer);
238 Error serialize_unpacked(uint32_t field_number, WriteBufferInterface& buffer)
const
243 const uint32_t size_x = this->
get_const(i).serialized_size();
252 return_value = this->
get_const(i).serialize(buffer);
259 Error deserialize_packed(ReadBufferInterface& buffer)
263 ReadBufferSection bufferSection(buffer, size);
266 return_value = x.deserialize(bufferSection);
269 return_value = this->
add(x);
272 return_value = x.deserialize(bufferSection);
285 Error deserialize_unpacked(ReadBufferInterface& buffer)
296 if(std::is_base_of<MessageInterface, DATA_TYPE>::value)
302 ReadBufferSection bufferSection(buffer, size);
303 return_value = this->
get(index).deserialize(bufferSection);
308 return_value = this->
get(index).deserialize(buffer);
324 #endif // End of _REPEATED_FIELD_H_
virtual Error set_data(const DATA_TYPE *data, const uint32_t length)=0
Given a different array of known length copy that data into this object.
This class is used in a message to calculate the current serialized size.
Definition: MessageSizeCalculator.h:50
Error serialize_with_id(uint32_t field_number, WriteBufferInterface &buffer) const final
Definition: RepeatedField.h:136
virtual Error add(const DATA_TYPE &value)=0
Append a value to the end of the array.
The pure virtual definition of a message buffer to read from.
Definition: ReadBufferInterface.h:43
Class template that specifies the interface of an arry with the data type.
Definition: RepeatedField.h:50
virtual uint32_t get_length() const =0
Obtain the total number of DATA_TYPE items in the array.
virtual uint32_t get_size() const =0
Obtain the total number of bytes currently stored in the array.
virtual uint32_t get_max_size() const =0
Obtain the maximum number of bytes which can at most be stored in the array.
Error deserialize(::EmbeddedProto::ReadBufferInterface &buffer) final
Function to deserialize this array.
Definition: RepeatedField.h:189
virtual const DATA_TYPE & get_const(uint32_t index) const =0
Get a constant reference to the value at the given index.
virtual ~RepeatedField()=default
@ NO_ERRORS
No errors have occurred.
The pure virtual definition of a message buffer used for writing .
Definition: WriteBufferInterface.h:46
Error
This enumeration defines errors which can occur during serialization and deserialization.
Definition: Errors.h:38
virtual void clear()=0
Remove all data in the array and set it to the default value.
@ END_OF_BUFFER
While trying to read from the buffer we ran out of bytes tor read.
virtual DATA_TYPE & get(uint32_t index)=0
Get a reference to the value at the given index.
uint32_t serialized_size_unpacked(int32_t field_number) const
Calculate the size of this field when serialized.
Definition: RepeatedField.h:219
DATA_TYPE & operator[](uint32_t index)
Get a reference to the value at the given index.
Definition: RepeatedField.h:95
@ ARRAY_FULL
The array is full, it is not possible to push more items in it.
Error serialize(WriteBufferInterface &buffer) const
Definition: RepeatedField.h:129
uint32_t get_size() const override
Obtain the total number of bytes currently stored in the buffer.
Definition: MessageSizeCalculator.h:63
virtual uint32_t get_max_length() const =0
Obtain the maximum number of DATA_TYPE items which can at most be stored in the array.
uint32_t serialized_size_packed(int32_t field_number) const
Calculate the size of this field when serialized.
Definition: RepeatedField.h:208
virtual void set(uint32_t index, const DATA_TYPE &value)=0
Set the value at the given index.
const DATA_TYPE & operator[](uint32_t index) const
Get a reference to the value at the given index. But constant.
Definition: RepeatedField.h:102
@ BUFFER_FULL
The write buffer is full, unable to push more bytes in to it.