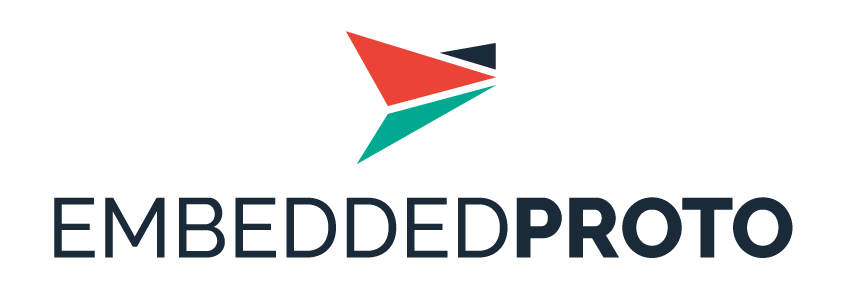 |
EmbeddedProto
2.0.0
EmbeddedProto is a C++ Protocol Buffer implementation specifically suitable for microcontrollers.
|
Go to the documentation of this file.
31 #ifndef _READ_BUFFER_SECTION_H_
32 #define _READ_BUFFER_SECTION_H_
79 bool peek(uint8_t&
byte)
const override;
91 void advance(
const uint32_t N)
override;
97 bool pop(uint8_t&
byte)
override;
108 const uint32_t max_size_;
113 #endif // _READ_BUFFER_SECTION_H_
ReadBufferSection()=delete
Explicitly delete the default constructor in favor of the one with parameters.
void advance() override
Decrement the size and call advance on the parent buffer.
Definition: ReadBufferSection.cpp:67
The pure virtual definition of a message buffer to read from.
Definition: ReadBufferInterface.h:43
uint32_t get_max_size() const override
Obtain the total number of bytes which can at most be stored in the buffer.
Definition: ReadBufferSection.cpp:52
bool peek(uint8_t &byte) const override
Expose the function of the parent buffer.
Definition: ReadBufferSection.cpp:57
virtual ~ReadBufferSection()=default
This is a wrapper around a ReadBufferInterface only exposing a given number of bytes.
Definition: ReadBufferSection.h:49
bool pop(uint8_t &byte) override
Decrement the size and pop the next byte from the parent buffer.
Definition: ReadBufferSection.cpp:86
uint32_t get_size() const override
Return the number of bytes remaining.
Definition: ReadBufferSection.cpp:47