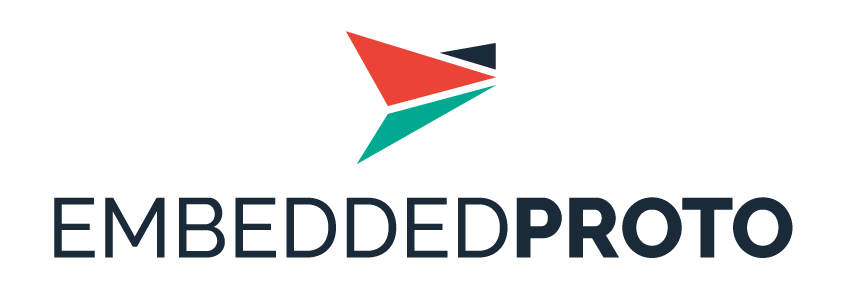 |
EmbeddedProto
2.0.0
EmbeddedProto is a C++ Protocol Buffer implementation specifically suitable for microcontrollers.
|
Go to the documentation of this file.
31 #ifndef _MESSAGE_SIZE_CALCULATOR_H_
32 #define _MESSAGE_SIZE_CALCULATOR_H_
71 return std::numeric_limits<uint32_t>::max();
77 return std::numeric_limits<uint32_t>::max();
81 bool push(
const uint8_t
byte)
override
90 bool push(
const uint8_t* bytes,
const uint32_t length)
override
This class is used in a message to calculate the current serialized size.
Definition: MessageSizeCalculator.h:50
~MessageSizeCalculator() override=default
uint32_t get_available_size() const override
To continue serialization return the maximum number that fits in a 32bit unsigned int.
Definition: MessageSizeCalculator.h:75
uint32_t get_max_size() const override
To continue serialization return the maximum number that fits in a 32bit unsigned int.
Definition: MessageSizeCalculator.h:69
void clear() override
Reset the size count of the buffer.
Definition: MessageSizeCalculator.h:57
The pure virtual definition of a message buffer used for writing .
Definition: WriteBufferInterface.h:46
bool push(const uint8_t byte) override
For calculating the size we just increment the counter and always return true.
Definition: MessageSizeCalculator.h:81
uint32_t get_size() const override
Obtain the total number of bytes currently stored in the buffer.
Definition: MessageSizeCalculator.h:63
MessageSizeCalculator()=default
bool push(const uint8_t *bytes, const uint32_t length) override
Increment the size with the given length.
Definition: MessageSizeCalculator.h:90